How to Read a Txt File in Python
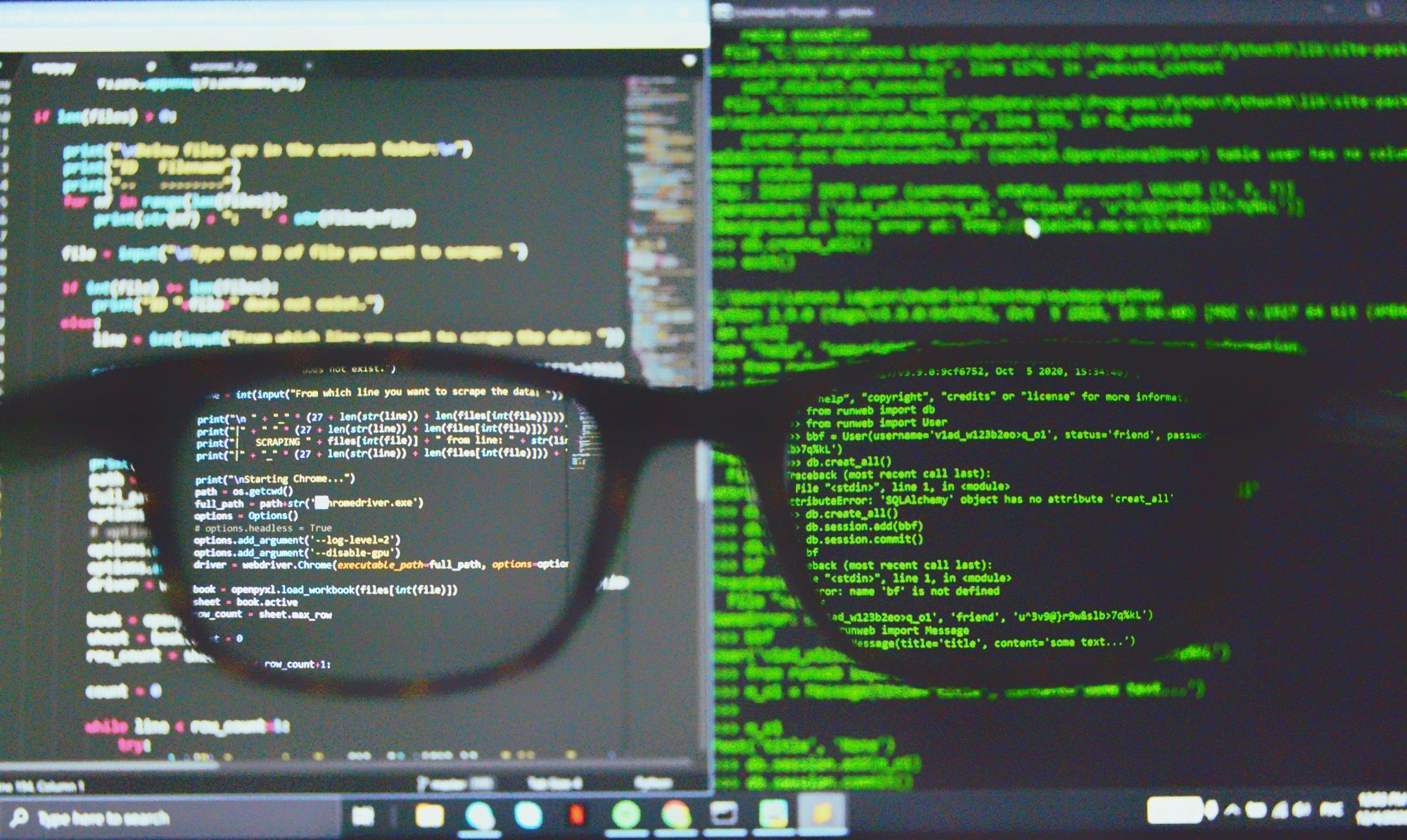
In Python, there are a few ways you can read a text file.
In this article, I volition go over the open()
part, the read()
, readline()
, readlines()
, close()
methods, and the with
keyword.
What is the open() function in Python?
If you lot desire to read a text file in Python, you start accept to open it.
This is the bones syntax for Python's open()
part:
open("name of file you want opened", "optional mode")
File names and right paths
If the text file and your current file are in the aforementioned directory ("binder"), then you can only reference the file name in the open()
function.
open("demo.txt")
Here is an case of both files being in the same directory:
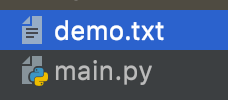
If your text file is in a different directory, then yous will need to reference the correct path name for the text file.
In this instance, the random-text
file is inside a unlike folder and then main.py
:
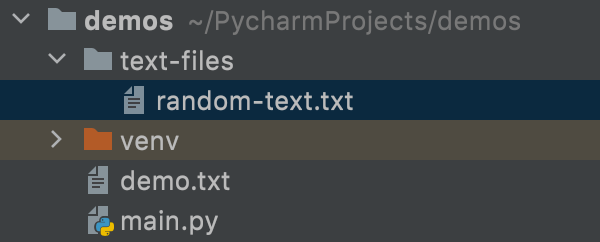
In lodge to admission that file in the master.py
, you have to include the binder proper noun with the name of the file.
open("text-files/random-text.txt")
If you don't have the right path for the file, and then you will get an mistake message like this:
open up("random-text.txt")

It is really important to go along track of which directory you are in so you can reference the correct path proper noun.
Optional Mode parameter in open()
There are unlike modes when you are working with files. The default mode is the read mode.
The letter r
stands for read manner.
open up("demo.txt", mode="r")
You can also omit mode=
and merely write "r"
.
open("demo.txt", "r")
In that location are other types of modes such every bit "westward"
for writing or "a"
for appending. I am non going to become into detail for the other modes because we are but going to focus on reading files.
For a complete list of the other modes, delight read through the documentation.
Additional parameters for the open()
part in Python
The open()
function can take in these optional parameters.
- buffering
- encoding
- errors
- newline
- closefd
- opener
To learn more about these optional parameters, delight read through the documentation.
What is the readable() method in Python?
If you want to bank check if a file can exist read, then you can use the readable()
method. This will return a True
or Simulated
.
This example would return True
because we are in the read mode:
file = open("demo.txt") print(file.readable())
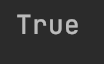
If I inverse this example, to "w"
(write) fashion, then the readable()
method would return False
:
file = open("demo.txt", "w") print(file.readable())
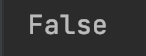
What is the read() method in Python?
The read()
method is going to read all of the content of the file equally one cord. This is a expert method to use if you don't have a lot of content in the text file.
In this instance, I am using the read()
method to impress out a list of names from the demo.txt
file:
file = open up("demo.txt") impress(file.read())

This method can accept in an optional parameter chosen size. Instead of reading the whole file, only a portion of it will be read.
If nosotros modify the earlier example, nosotros can print out only the first word by adding the number 4 as an statement for read()
.
file = open("demo.txt") impress(file.read(iv))

If the size argument is omitted, or if the number is negative, and then the whole file will exist read.
What is the close() method in Python?
Once you are washed reading a file, information technology is of import that y'all close it. If you lot forget to shut your file, then that tin crusade bug.
This is an example of how to close the demo.txt
file:
file = open up("demo.txt") print(file.read()) file.shut()
How to use the with
keyword to close files in Python
One way to ensure that your file is closed is to utilize the with
keyword. This is considered skilful practice, because the file will shut automatically instead of you having to manually close information technology.
Hither is how to rewrite our case using the with
keyword:
with open("demo.txt") as file: print(file.read())
What is the readline() method in Python?
This method is going to read i line from the file and return that.
In this example, nosotros have a text file with these two sentences:
This is the first line This is the second line
If nosotros use the readline()
method, it will only impress the first sentence of the file.
with open("demo.txt") as file: print(file.readline())

This method also takes in the optional size parameter. We can modify the example to add the number vii to only read and print out This is
:
with open("demo.txt") as file: print(file.readline(7))
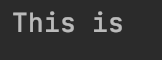
What is the readlines() method in Python?
This method volition read and render a listing of all of the lines in the file.
In this example, we are going to impress out our grocery items every bit a list using the readlines()
method.
with open("demo.txt") as file: print(file.readlines())

How to use a for loop to read lines from a file in Python
An alternative to these unlike read methods would be to apply a for loop
.
In this case, nosotros can print out all of the items in the demo.txt
file by looping over the object.
with open("demo.txt") as file: for particular in file: impress(detail)

Conclusion
If you desire to read a text file in Python, you start have to open information technology.
open("name of file you want opened", "optional mode")
If the text file and your current file are in the aforementioned directory ("binder"), then you tin just reference the file proper name in the open up()
office.
If your text file is in a different directory, and so you will demand to reference the right path name for the text file.
The open()
function takes in the optional mode parameter. The default style is the read manner.
open("demo.txt", "r")
If y'all want to check if a file can be read, then you can employ the readable()
method. This volition return a True
or False
.
file.readable()
The read()
method is going to read all of the content of the file as one string.
file.read()
In one case you lot are washed reading a file, it is important that yous close it. If you forget to close your file, then that tin can cause issues.
file.close()
Ane manner to ensure that your file is airtight is to utilise the with
keyword.
with open up("demo.txt") as file: impress(file.read())
The readline()
method is going to read one line from the file and render that.
file.readline()
The readlines()
method will read and render a listing of all of the lines in the file.
file.readlines()
An alternative to these dissimilar read methods would be to use a for loop
.
with open("demo.txt") as file: for item in file: print(particular)
I promise yous enjoyed this article and best of luck on your Python journey.
Learn to lawmaking for free. freeCodeCamp'due south open source curriculum has helped more than than xl,000 people get jobs equally developers. Get started
Source: https://www.freecodecamp.org/news/python-open-file-how-to-read-a-text-file-line-by-line/
0 Response to "How to Read a Txt File in Python"
Post a Comment